Hello, guys in this tutorial we will create an animated pure CSS toggle switch ( ON-OFF Switch ) simple checkbox using HTML & CSS
Hello Friends, Today in this post, we’ll find out how to Create a Custom ON/OFF switch Button. recently I’ve got shared make a Custom Checkbox Design Using HTML & CSS, but our today’s topic could be a Create a CSS Toggle Switch.
Welcome to an another article on how to create an awesome Toggle Switch button with CSS. Are those “default checkboxes” too boring for you? Want to make a much better ON/OFF toggle button? although we cannot use direct CSS to customize it, you can still do it pretty easily with just some lines of HTML and CSS.
In order to make toggle switch, we’re going to be using traditional elements but they’re going to be hidden through CSS. Our CSS will then make use of the: checked pseudo-class and the general sibling combinator (~) to dynamically apply either an “on” or “off” style to our switch button.
Let’s start with the HTML, we’re going to be wrapping everything inside an element. This will allow us to click anywhere within the wrapper and it will toggle the state of our hidden checkbox.
Understanding CSS Toggle Switches
Before we dive into creating CSS toggle switches, it’s important to understand the basics. A toggle switch is an input element that allows users to switch between two states. When the toggle switch is in the “on” state, it displays one set of content or performs one action, and when it’s in the “off” state, it displays another set of content or performs a different action.
To create a toggle switch, you will need to use HTML and CSS. The HTML will define the structure of the toggle switch, and the CSS will define its appearance and behavior.
How to Create a html CSS toggle switch button Step by Step
Step 1 — Creating a New Project
The first thing we’ll do is create a folder that will contain all of the files that make up the project. Create an empty folder on your devices and name it “as you want”.
Open up Visual Studio Code or any Text editor which is you liked, and create files(index.html, style.css) inside the folder which you have created for toggle button. In the next step, we will start creating the basic structure of the webpage.
Step 2 — Setting Up the basic structure
In this step, we will add the HTML code to create the basic structure of the project.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <title>ON-OFF Switch Button</title> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <meta https-equiv="X-UA-Compatible" content="ie=edge" /> <link rel="stylesheet" href="style.css" /> <link href="https://fonts.googleapis.com/css2?family=IBM+Plex+Sans:wght@500&display=swap" rel="stylesheet"> </head> <body> </body> </html>
This is the base structure of most web pages that use HTML.
Add the following code inside the <body>
tag:
<div class="switch-toggle"> <div class="button-check" id="button-check"> <input type="checkbox" class="checkbox"> <span class="switch-btn"></span> <span class="layer"></span> </div> </div>
Step 3 — Adding Styles for the Classes
In this step, we will add CSS code for style our html elements.
* { padding: 0; margin: 0; outline: 0; font-family: 'IBM Plex Sans', sans-serif; } body { display: flex; align-items: center; justify-content: center; height: 100vh; width: 100vw; overflow: hidden; } .switch-toggle { display: flex; height: 100%; align-items: center; } .switch-btn, .layer { position: absolute; top: 0; right: 0; bottom: 0; left: 0; } .button-check { position: relative; width: 90px; height: 46px; overflow: hidden; border-radius: 50px; -webkit-border-radius: 50px; -moz-border-radius: 50px; -ms-border-radius: 50px; -o-border-radius: 50px; } .checkbox { position: relative; width: 100%; height: 100%; padding: 0; margin: 0; opacity: 0; cursor: pointer; z-index: 3; } .switch-btn { z-index: 2; } .layer { width: 100%; background-color: #8cf7a0; transition: 0.3s ease all; z-index: 1; } #button-check .switch-btn:before, #button-check .switch-btn:after { position: absolute; top: 4px; left: 4px; width: 30px; height: 20px; color: #fff; font-size: 10px; font-weight: bold; text-align: center; line-height: 1; padding: 9px 4px; background-color: #00921c; border-radius: 50%; transition: 0.3s cubic-bezier(0.18, 0.89, 0.35, 1.15) all; display: flex; align-items: center; justify-content: center; } #button-check .switch-btn:before { content: 'ON'; } #button-check .switch-btn:after { content: 'OFF'; } #button-check .switch-btn:after { right: -50px; left: auto; background-color: #F44336; } #button-check .checkbox:checked + .switch-btn:before { left: -50px; } #button-check .checkbox:checked + .switch-btn:after { right: 4px; } #button-check .checkbox:checked ~ .layer { background-color: #fdd1d1; }
#Final Result
How to create a toggle switch using JavaScript?
Step 1: Creating the HTML Structure
The first step in creating a toggle switch is to create the HTML structure. Here’s an example of what the HTML structure for a toggle switch might look like:
<div class="toggle-switch"> <input type="checkbox" id="toggle" class="toggle-switch-checkbox"> <label for="toggle" class="toggle-switch-label"></label> </div>
Step 2: Styling the Toggle Switch
Now that we have the HTML structure in place, it’s time to add some styles. Here’s an example of what the CSS for a toggle switch might look like:
.toggle-switch { position: relative; display: inline-block; width: 60px; height: 34px; } .toggle-switch-checkbox { display: none; } .toggle-switch-label { position: absolute; cursor: pointer; top: 0; left: 0; right: 0; bottom: 0; background-color: #ccc; -webkit-transition: .4s; transition: .4s; border-radius: 34px; } .toggle-switch-label:before { position: absolute; content: ""; height: 26px; width: 26px; left: 4px; bottom: 4px; background-color: white; -webkit-transition: .4s; transition: .4s; border-radius: 50%; } .toggle-switch-checkbox:checked + .toggle-switch-label { background-color: #2196F3; } .toggle-switch-checkbox:checked + .toggle-switch-label:before { -webkit-transform: translateX(26px); -ms-transform: translateX(26px); transform: translateX(26px); background-color: #2196F3; }
Step 3: Adding Functionality with Javascript
Now that we have the HTML and CSS in place, it’s time to add some functionality using Javascript. Here’s an example of what the Javascript code for a toggle switch might look like:
const toggleSwitch = document.querySelector('.toggle-switch-checkbox'); function switchTheme(event) { if (event.target.checked) { document.documentElement.setAttribute('data-theme', 'dark'); } else { document.documentElement.setAttribute('data-theme', 'light'); } } toggleSwitch.addEventListener('change', switchTheme, false);
Let’s break down what this code does:
- The
querySelector
method is used to select theinput
element with the classtoggle-switch-checkbox
. - The
switchTheme
function is defined to toggle the theme when the toggle switch is clicked. - Inside the
switchTheme
function, anif
statement checks whether the toggle switch is checked or not. If it is checked, thedata-theme
attribute of thehtml
element is set todark
. If it is not checked, thedata-theme
attribute of thehtml
element is set tolight
. - The
addEventListener
method is used to add an event listener to theinput
element. When thechange
event is triggered (i.e., the toggle switch is clicked), theswitchTheme
function is called.
#final result
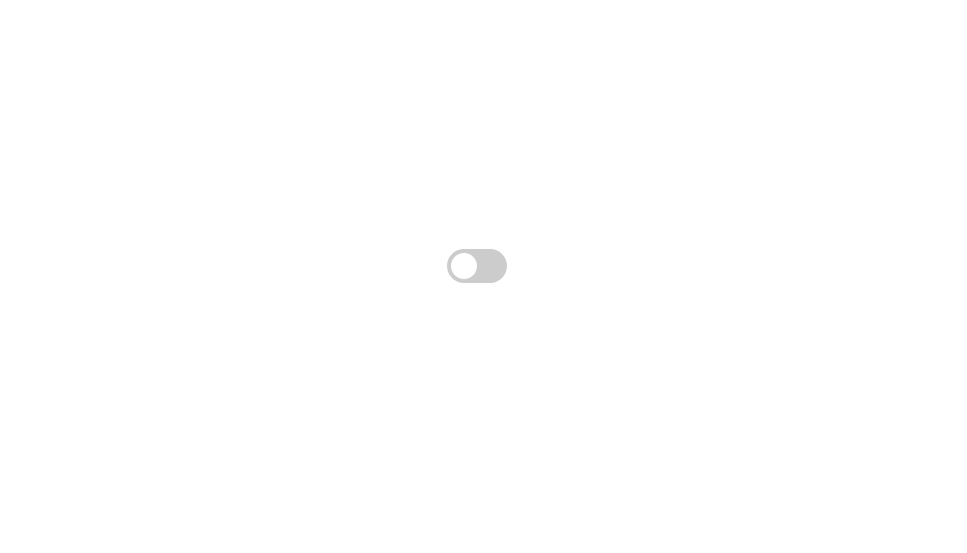
list of the 20 best CSS toggle switch buttons
#1 CSS Toggle Button (Checkbox Hack)
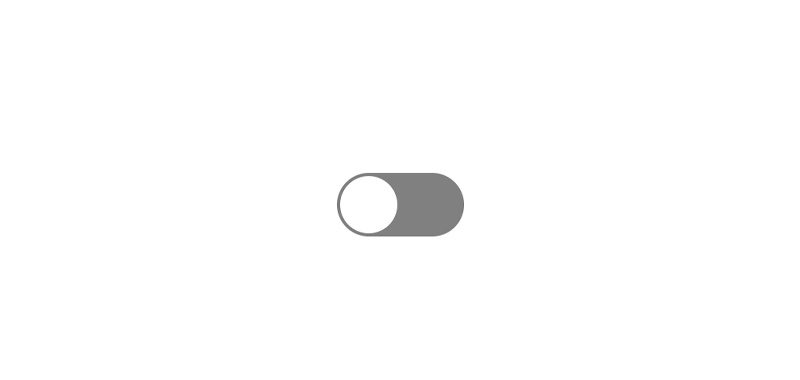
CSS Toggle Button (Checkbox Hack), which was developed by Marcus Burnette. Moreover, you can customize it according to your wish and need.
Author: | Marcus Burnette |
Created on: | January 12, 2017 |
Made with: | HTML & CSS(SCSS) |
Demo Link: | Source Code / Demo |
Tags: | CSS Toggle Switch (Checkbox Hack) |
#2 Simple CSS toggle on/off button
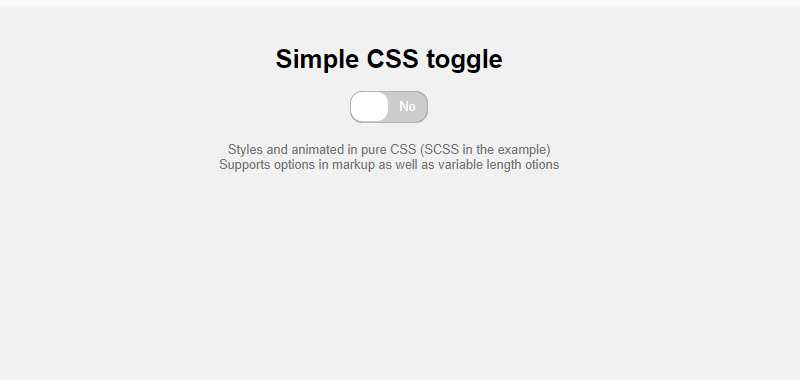
Simple CSS toggle on/off button, which was developed by Tim Wickstrom. Moreover, you can customize it according to your wish and need.
Author: | Tim Wickstrom |
Created on: | May 14, 2014 |
Made with: | HTML & CSS(SCSS) |
Demo Link: | Source Code / Demo |
Tags: | Simple CSS toggle switch |
#3 Pure CSS3 Toggle Switch
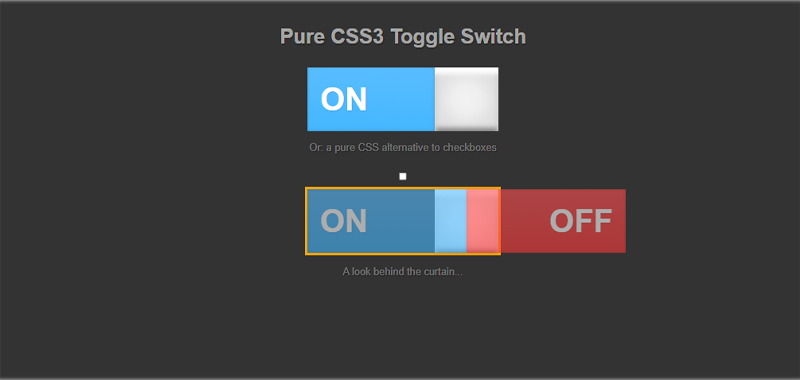
Pure CSS3 Toggle Switch, which was developed by Andy McFee. Moreover, you can customize it according to your wish and need.
Author: | Andy McFee |
Created on: | July 28, 2012 |
Made with: | HTML & CSS |
Demo Link: | Source Code / Demo |
Tags: | Pure CSS3 Toggle Switch |
#4 Single Element Pure CSS Toggle Button
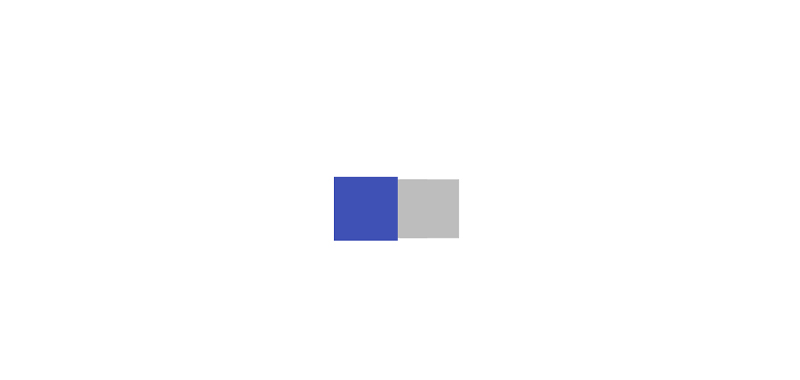
Single Element Pure CSS Toggle Button, which was developed by ari. Moreover, you can customize it according to your wish and need.
Author: | ari |
Created on: | February 25, 2016 |
Made with: | HTML & CSS(SCSS) |
Demo Link: | Source Code / Demo |
Tags: | Single Element CSS Toggle |
#5 Awesome toggle switch button
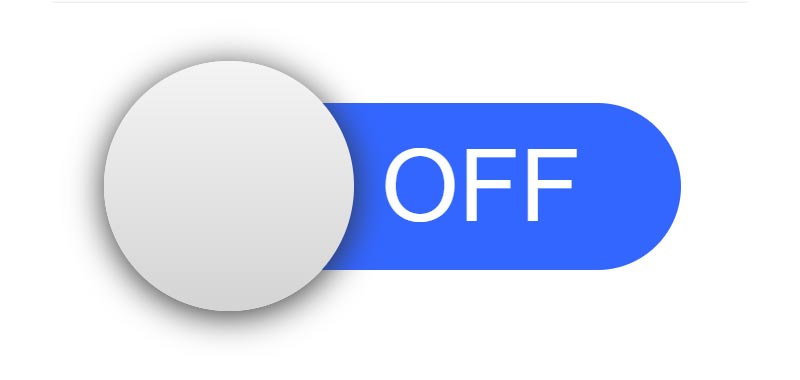
Awesome toggle switch button, which was developed by Stephen Tudor. Moreover, you can customize it according to your wish and need.
Author: | Stephen Tudor |
Created on: | September 6, 2012 |
Made with: | HTML & CSS(SCSS) & JS |
Demo Link: | Source Code / Demo |
Tags: | Awesome toggle switch button |
#6 Dark Mode Toggle Button
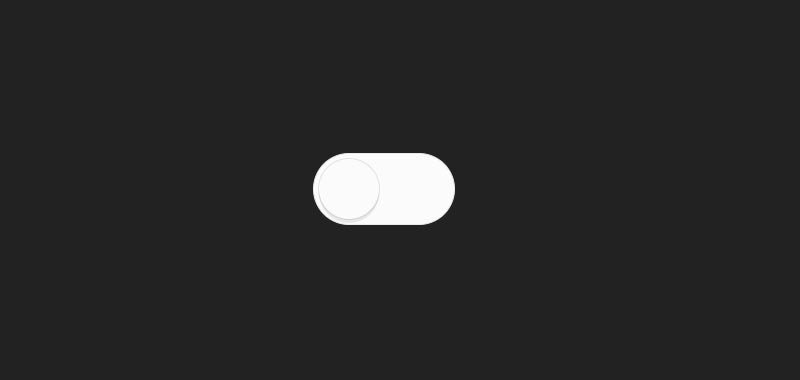
Dark Mode Toggle, which was developed by James. Moreover, you can customize it according to your wish and need.
Author: | James |
Created on: | September 19, 2019 |
Made with: | HTML & CSS & JS |
Demo Link: | Source Code / Demo |
Tags: | Dark Mode Toggle |
#7 Only CSS: Toggle Switch
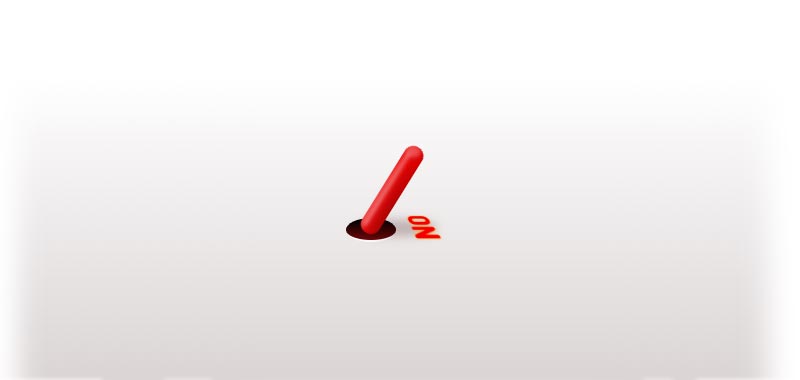
Only CSS: Toggle Switch, which was developed by Yusuke Nakaya. Moreover, you can customize it according to your wish and need.
Author: | Yusuke Nakaya |
Created on: | July 4, 2017 |
Made with: | HTML & CSS & JS |
Demo Link: | Source Code / Demo |
Tags: | Only CSS: Toggle Switch |
#8 Toggle switch using a checkbox input
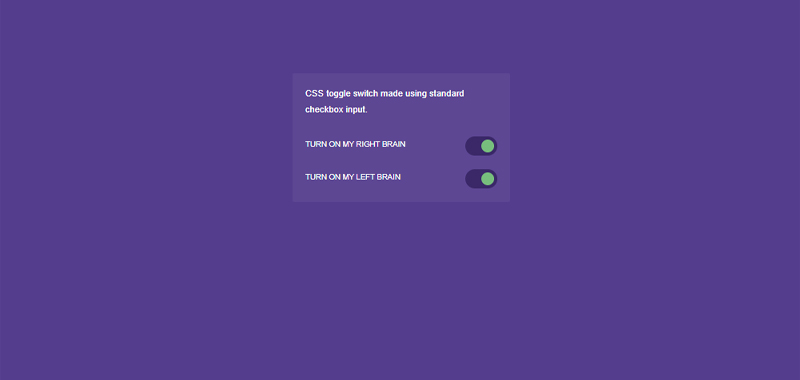
Toggle switch using a checkbox input, which was developed by Ketan Mistry. Moreover, you can customize it according to your wish and need.
Author: | Ketan Mistry |
Created on: | July 16, 2017 |
Made with: | HTML & CSS |
Demo Link: | Source Code / Demo |
Tags: | CSS toggle using a checkbox input |
#9 Artsy Slide Switches with Backgrounds
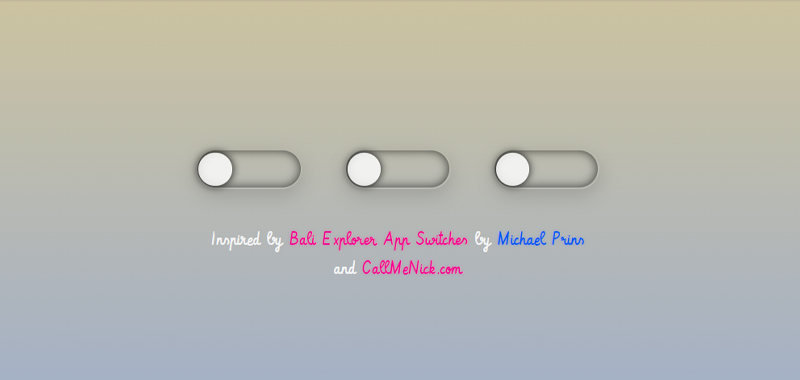
Artsy Slide Switches with Backgrounds, which was developed by Advait Junnarkar. Moreover, you can customize it according to your wish and need.
Author: | Advait Junnarkar |
Created on: | June 13, 2017 |
Made with: | HTML & CSS &JS |
Demo Link: | Source Code / Demo |
Tags: | Artsy Slide Switches with Backgrounds |
#10 Checkbox Toggle Button
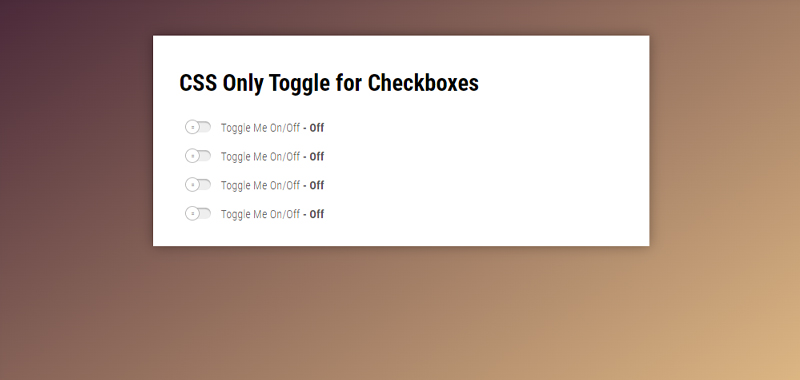
Checkbox Toggle Button, which was developed by Alexander Demchak. Moreover, you can customize it according to your wish and need.
Author: | Alexander Demchak |
Created on: | July 19, 2017 |
Made with: | HTML & CSS |
Demo Link: | Source Code / Demo |
Tags: | CSS Toggle Button |
#11 Skew Toggle Switch Button
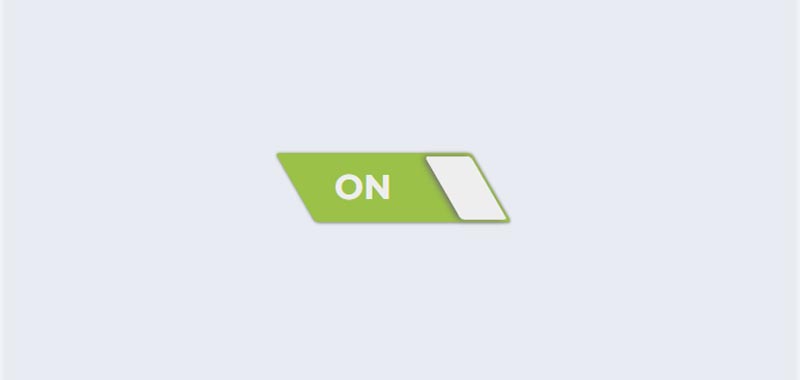
Skew Toggle Switch Button, which was developed by Abhijit Hota. Moreover, you can customize it according to your wish and need.
Author: | Abhijit Hota |
Created on: | December 16, 2014 |
Made with: | Haml, SCSS & JS |
Demo Link: | Source Code / Demo |
Tags: | Skew Toggle Switch Button |
#12 Pure CSS Day/Night Toggle Button
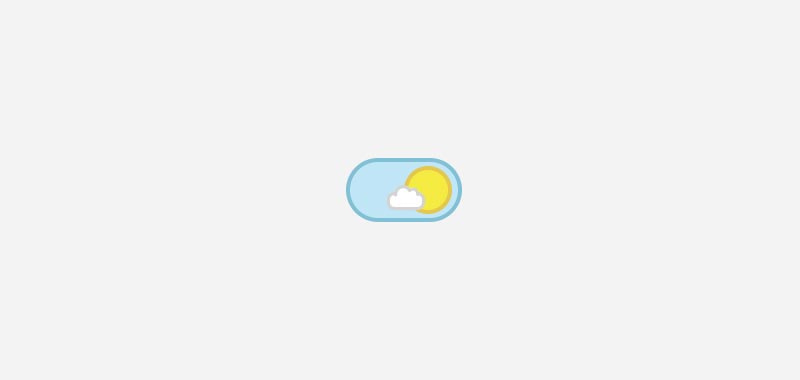
Pure CSS Day/Night Toggle Button, which was developed by Jason Tyler. Moreover, you can customize it according to your wish and need.
Author: | Jason Tyler |
Created on: | January 31, 2015 |
Made with: | HTML, SCSS & JS |
Demo Link: | Source Code / Demo |
Tags: | CSS Day/Night Toggle Button |
#13 Flat Toggle Switch Button

Flat Toggle Switch Button, which was developed by Felix De Montis. Moreover, you can customize it according to your wish and need.
Author: | Felix De Montis |
Created on: | September 5, 2013 |
Made with: | Haml, SCSS |
Demo Link: | Source Code / Demo |
Tags: | Flat Toggle Switch Button |
#14 Minimal Toggle Switch Button
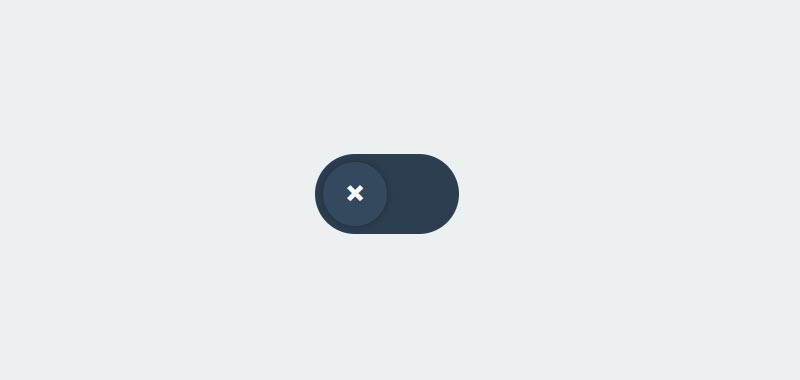
Minimal Toggle Switch Button, which was developed by Raúl Barrera. Moreover, you can customize it according to your wish and need.
Author: | Raúl Barrera |
Created on: | February 8, 2016 |
Made with: | HTML & SCSS |
Demo Link: | Source Code / Demo |
Tags: | Minimal Toggle Switch Button |
#15 Liquid Toggle button
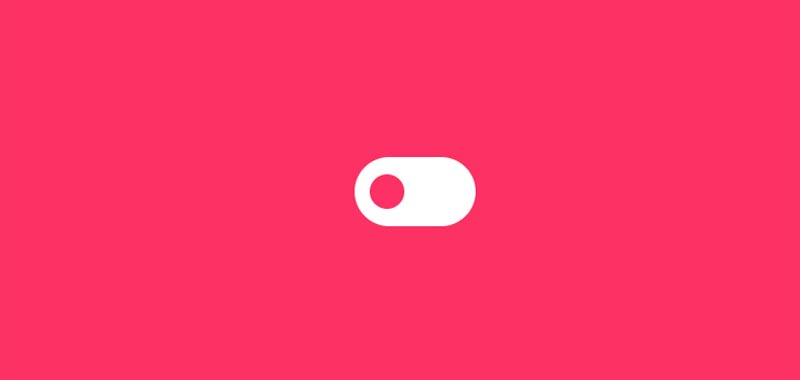
Liquid Toggle button, which was developed by Alex. Moreover, you can customize it according to your wish and need.
Author: | Alex |
Created on: | June 22, 2017 |
Made with: | HTML(Pug), SCSS & JS |
Demo Link: | Source Code / Demo |
Tags: | Liquid Toggle button |
#16 Light Bulb Toggle Switch Button
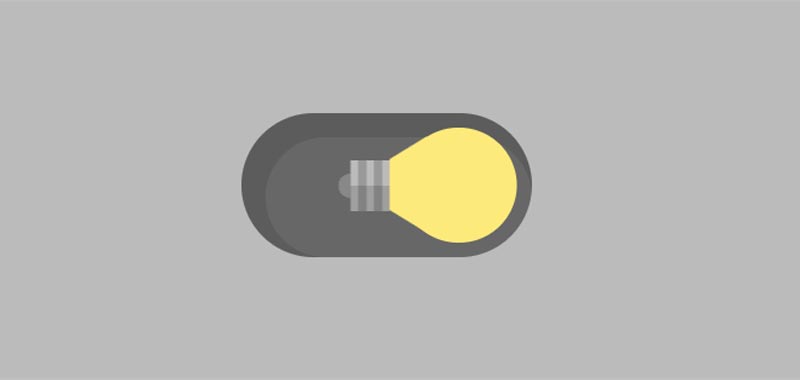
Light Bulb Toggle Switch Button, which was developed by Jon Kantner. Moreover, you can customize it according to your wish and need.
Author: | Jon Kantner |
Created on: | March 26, 2018 |
Made with: | HTML & CSS |
Demo Link: | Source Code / Demo |
Tags: | Light Bulb Toggle Switch Button |
#17 CSS 3D Toggle Switch
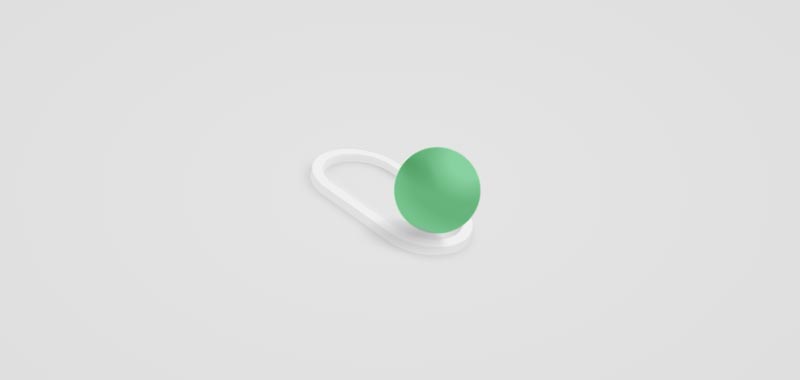
CSS 3D Toggle Switch, which was developed by Ana Tudor. Moreover, you can customize it according to your wish and need.
Author: | Ana Tudor |
Created on: | July 21, 2018 |
Made with: | HTML & CSS(SCSS) |
Demo Link: | Source Code / Demo |
Tags: | CSS 3D Toggle Switch |
#18 On-Off switch button
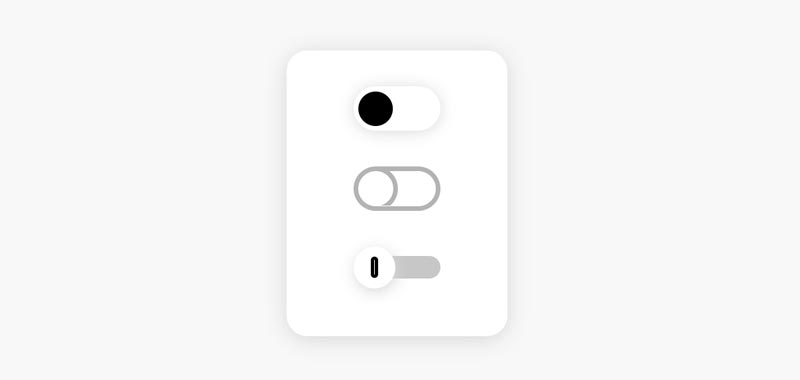
On-Off switch button, which was developed by Himalaya Singh. Moreover, you can customize it according to your wish and need.
Author: | Himalaya Singh |
Created on: | August 22, 2018 |
Made with: | HTML & CSS |
Demo Link: | Source Code / Demo |
Tags: | On-Off switch button |
#19 CSS toggle switch animation
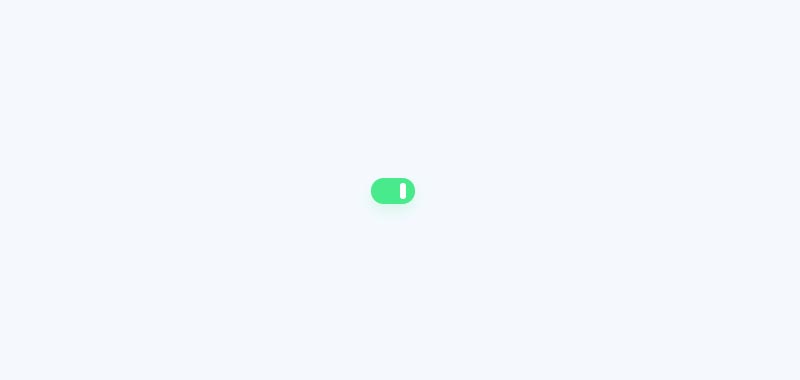
toggle switch animation, which was developed by Aaron Iker. Moreover, you can customize it according to your wish and need.
Author: | Aaron Iker |
Created on: | October 25, 2018 |
Made with: | HTML & CSS(SCSS) |
Demo Link: | Source Code / Demo |
Tags: | toggle switch animation |
#20 Pure CSS Gender Toggle Switch
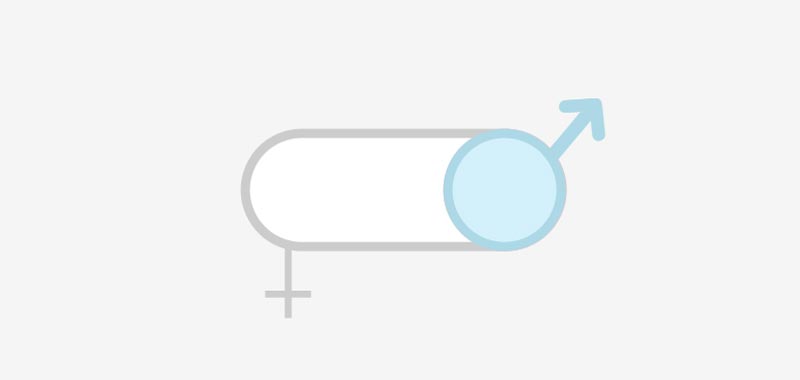
Pure CSS Gender Toggle Button, which was developed by Chintu Yadav Sara. Moreover, you can customize it according to your wish and need.
Author: | Chintu Yadav Sara |
Created on: | January 1, 2019 |
Made with: | HTML & CSS |
Demo Link: | Source Code / Demo |
Tags: | CSS Gender Toggle Button |
#21 Pure CSS Day Night toggle switch
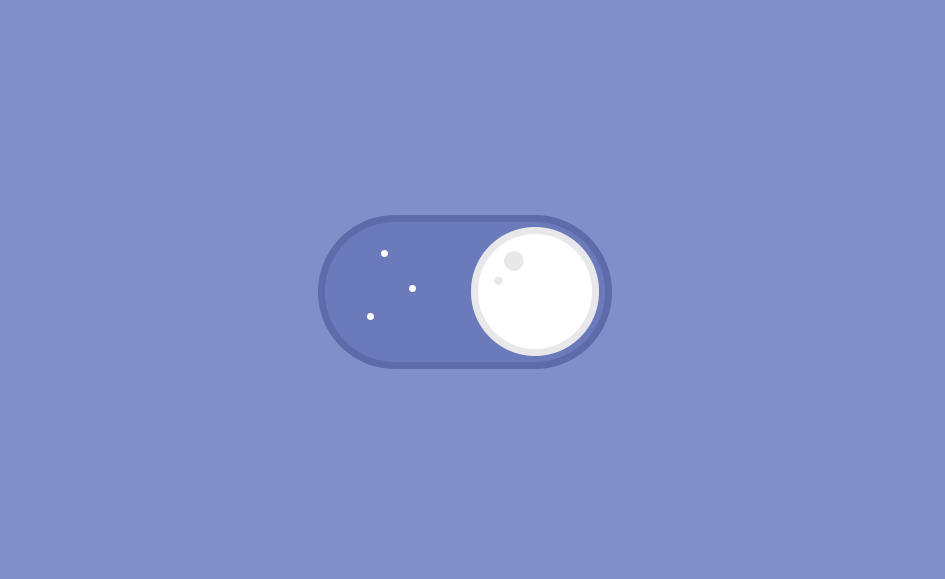
Pure CSS Day Night toggle switch, which was developed by Chris Bongers. Moreover, you can customize it according to your wish and need.
Author: | Chris Bongers |
Created on: | DECEMBER 23, 2020 |
Made with: | HTML & CSS |
Demo Link: | Source Code / Demo |
Tags: | Pure CSS Day Night toggle switch |
#22 Neon Light Toggle Button
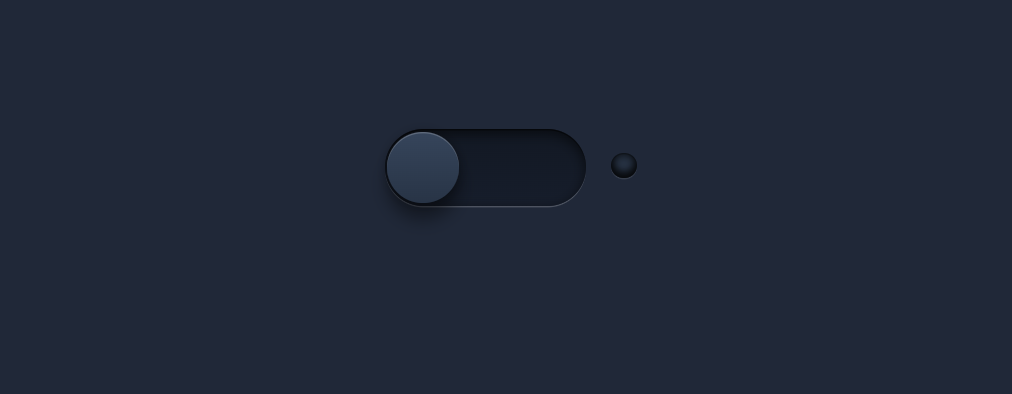
Neon Light Toggle Button, which was developed by Stef Geraets. Moreover, you can customize it according to your wish and need.
Author: | Stef Geraets |
Created on: | APRIL 9, 2014 |
Made with: | HTML & CSS |
Demo Link: | Source Code / Demo |
Tags: | Neon Light Toggle Switch |
#23 Day and Night Toggle Switch button
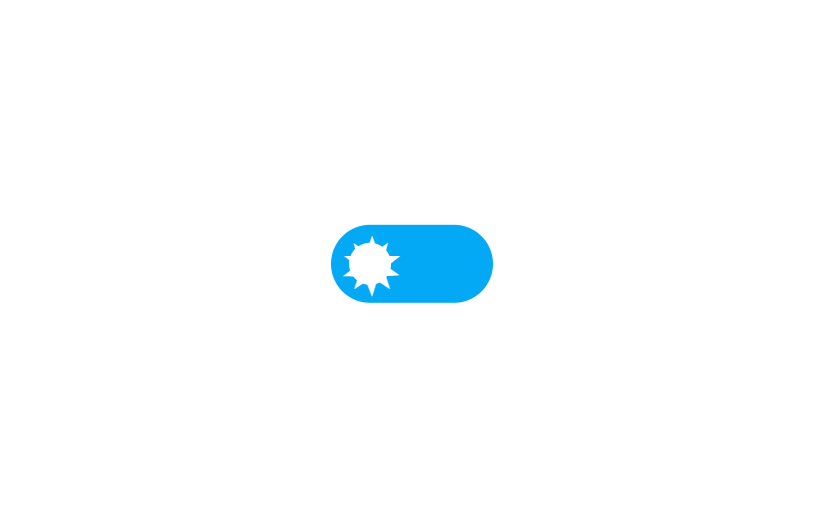
Day and Night Toggle Switch button, which was developed by Himalaya Singh. Moreover, you can customize it according to your wish and need.
Author: | Himalaya Singh |
Created on: | MAY 22, 2019 |
Made with: | HTML & CSS |
Demo Link: | Source Code / Demo |
Tags: | Day and Night Toggle Switch button |
#24 On/Off Toggle Switch button
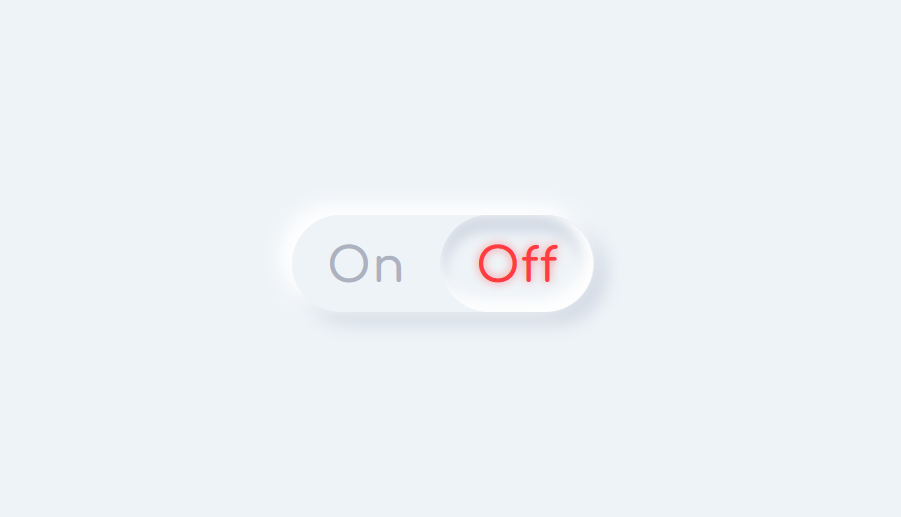
On/Off Toggle Switch button, which was developed by @BrawadaCom. Moreover, you can customize it according to your wish and need.
Author: | @BrawadaCom |
Created on: | APRIL 23, 2021 |
Made with: | HTML, CSS & JS |
Demo Link: | Source Code / Demo |
Tags: | On/Off Toggle Switch button |
#25 Airplane Mode Toggle Switch
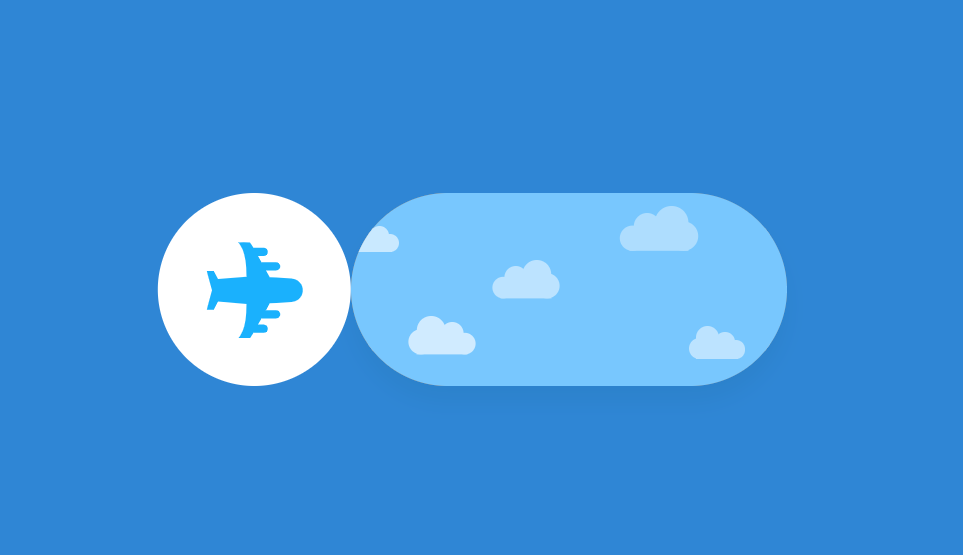
Airplane Mode Toggle Switch, which was developed by Kiarash Zarinmehr. Moreover, you can customize it according to your wish and need.
Author: | Kiarash Zarinmehr |
Created on: | JANUARY 27, 2020 |
Made with: | HTML, CSS & JS |
Demo Link: | Source Code / Demo |
Tags: | Airplane Mode Toggle Switch |
#26 Gender toggle switch
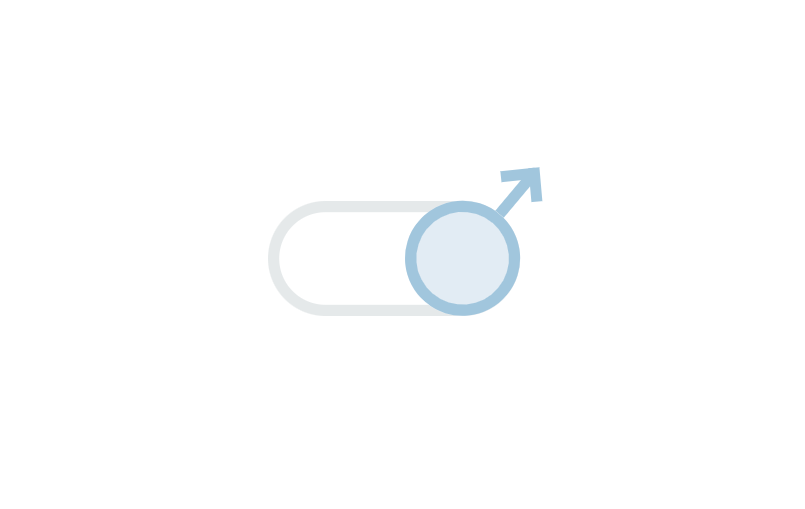
Gender toggle switch, which was developed by Mert Cukuren. Moreover, you can customize it according to your wish and need.
Author: | Mert Cukuren |
Created on: | JUNE 16, 2019 |
Made with: | HTML & CSS (SCSS) |
Demo Link: | Source Code / Demo |
Tags: | Gender toggle switch |
#27 Simple Toggle Switch Button
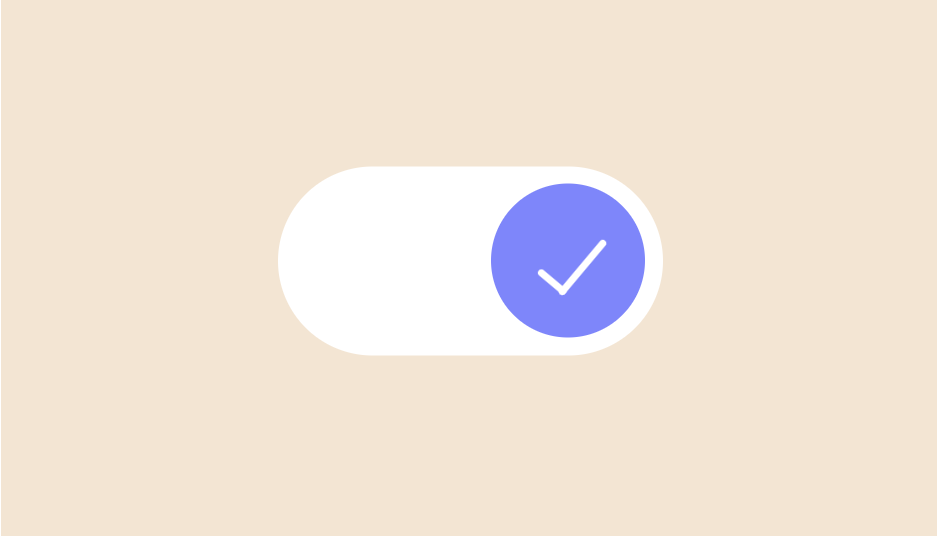
Simple Toggle Switch Button, which was developed by Kev_kan_code. Moreover, you can customize it according to your wish and need.
Author: | Kev_kan_code |
Created on: | APRIL 6, 2017 |
Made with: | HTML, CSS (SCSS) & JS |
Demo Link: | Source Code / Demo |
Tags: | Simple Toggle Switch Button |
#28 Tiny Toggle Switch
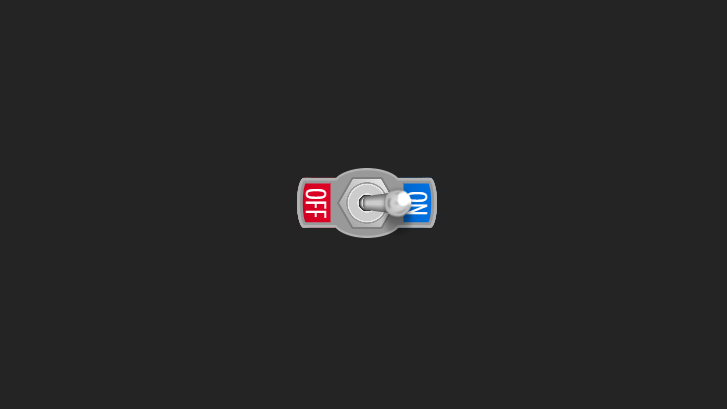
Tiny Toggle Switch, which was developed by Jon Kantner. Moreover, you can customize it according to your wish and need.
Author: | Jon Kantner |
Created on: | SEPTEMBER 6, 2019 |
Made with: | HTML, CSS |
Demo Link: | Source Code / Demo |
Tags: | Tiny Toggle Switch |
#29 Toy Toggle Switch
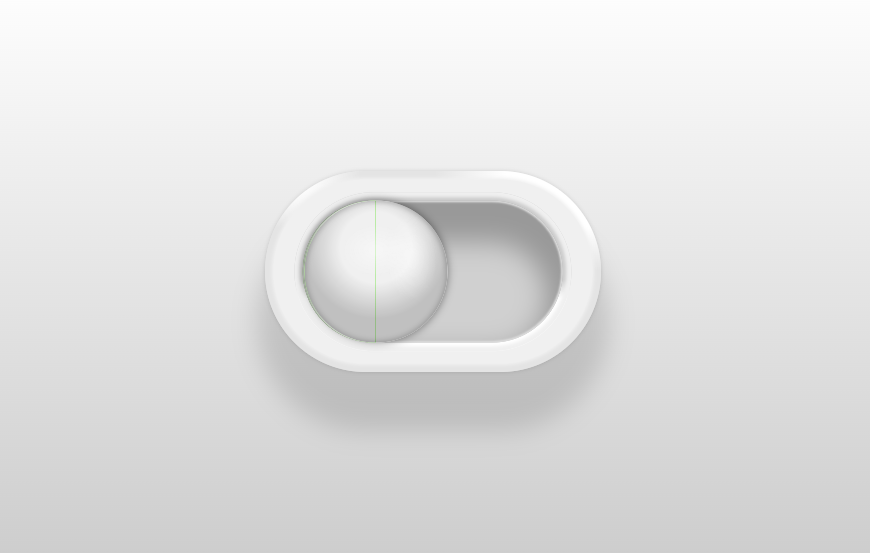
Toy Toggle Switch, which was developed by Jon Kantner. Moreover, you can customize it according to your wish and need.
Author: | Jon Kantner |
Created on: | JULY 2, 2019 |
Made with: | HTML, CSS |
Demo Link: | Source Code / Demo |
Tags: | Toy Toggle Switch |
#30 Simple CSS Switch / Toggle

Simple CSS Switch, which was developed by Aaron Iker. Moreover, you can customize it according to your wish and need.
Author: | Aaron Iker |
Created on: | OCTOBER 17, 2019 |
Made with: | HTML, CSS (SCSS) |
Demo Link: | Source Code / Demo |
Tags: | Simple CSS Switch |
#31 Awesome Toggle Button
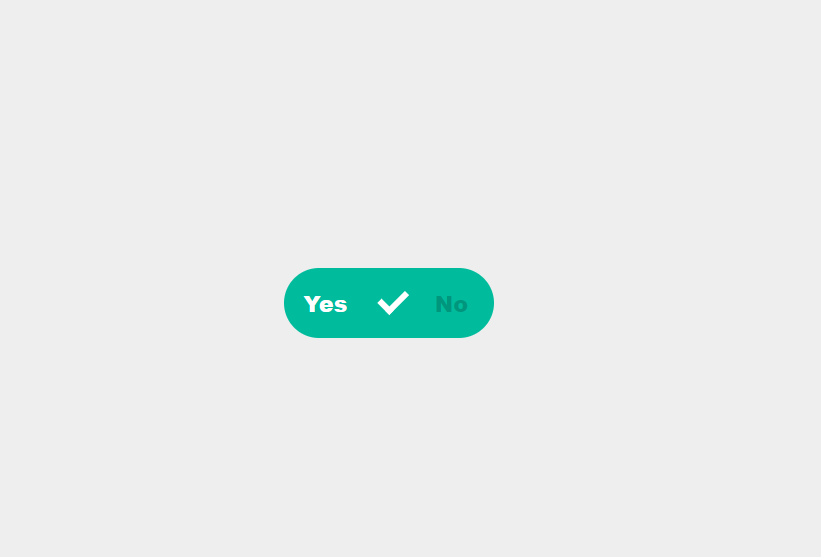
Awesome Toggle Button, which was developed by Andrew. Moreover, you can customize it according to your wish and need.
Author: | Andrew |
Created on: | OCTOBER 7, 2014 |
Made with: | HTML, CSS |
Demo Link: | Source Code / Demo |
Tags: | Awesome Toggle Button |
#32 Sleek Sliding Toggle Checkbox
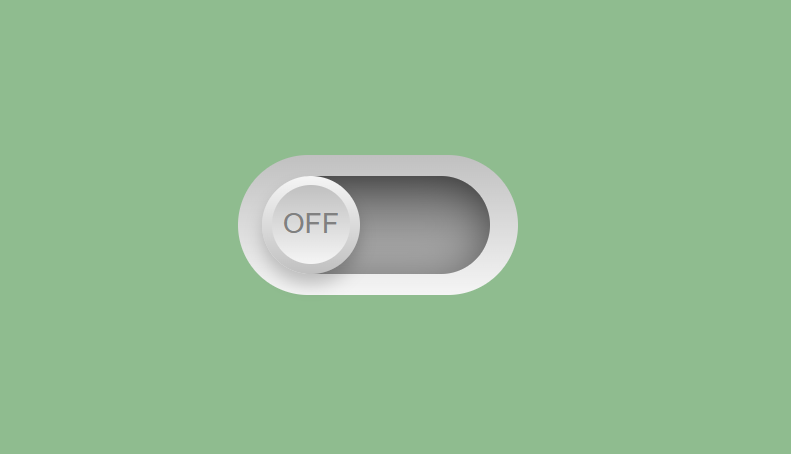
Sleek Sliding Toggle Checkbox, which was developed by Comehope. Moreover, you can customize it according to your wish and need.
Author: | Comehope |
Created on: | APRIL 27, 2018 |
Made with: | HTML, CSS |
Demo Link: | Source Code / Demo |
Tags: | Sleek Sliding Toggle Checkbox |
#33 Skeuomorphic Toggle Switch
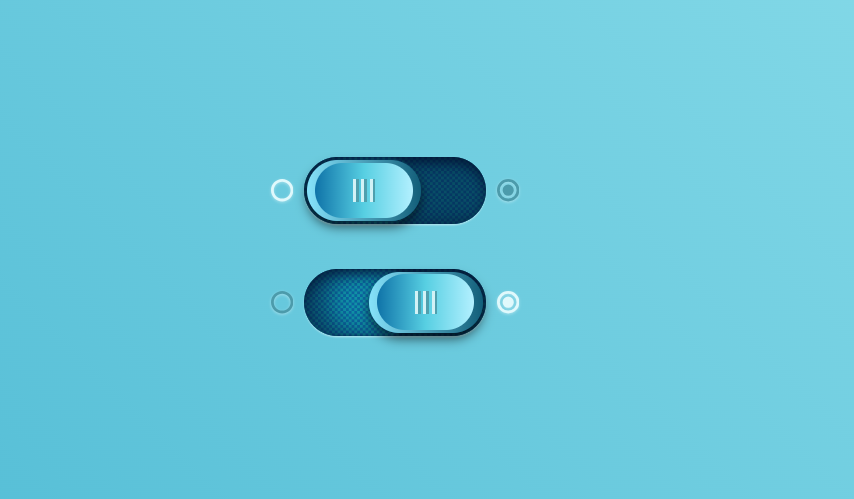
Skeuomorphic Toggle Switch, which was developed by Nicolas Jesenberger. Moreover, you can customize it according to your wish and need.
Author: | Nicolas Jesenberger |
Created on: | MAY 7, 2023 |
Made with: | HTML, CSS (SCSS) |
Demo Link: | Source Code / Demo |
Tags: | Skeuomorphic Toggle Switch |
#34 Red Dot toggle switch
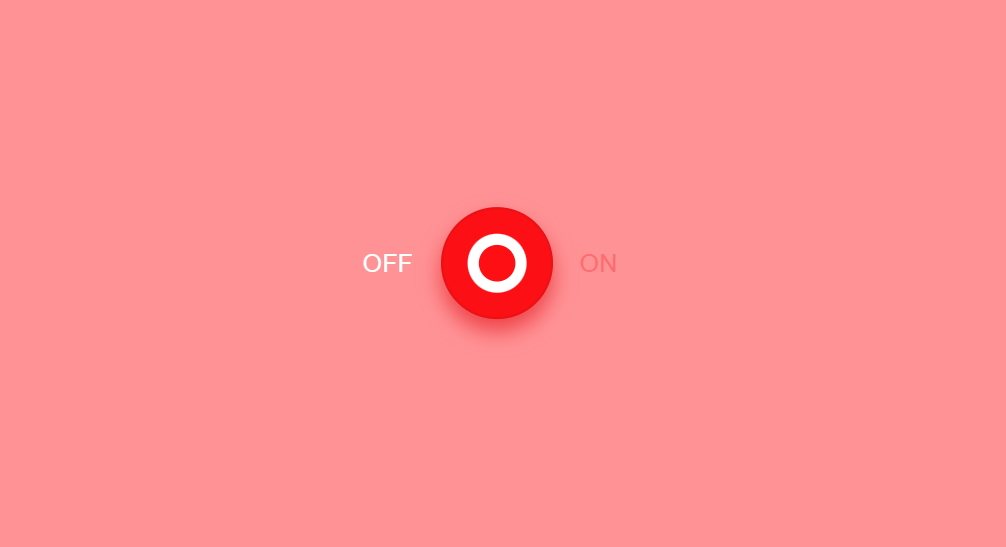
Red Dot toggle switch, which was developed by Anastasia Goodwin. Moreover, you can customize it according to your wish and need.
Author: | Anastasia Goodwin |
Created on: | AUGUST 3, 2018 |
Made with: | HTML, CSS (SCSS) & JS |
Demo Link: | Source Code / Demo |
Tags: | Red Dot toggle switch |
#35 Checkbox Rocker Toggle Switch
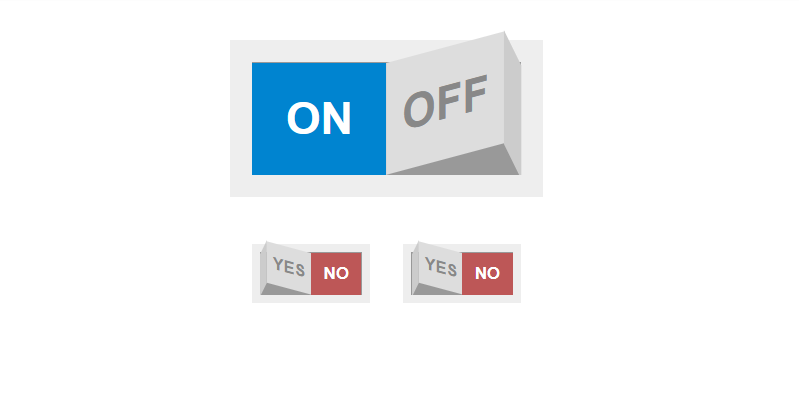
Checkbox Rocker Toggle Switch, which was developed by Marcus Connor. Moreover, you can customize it according to your wish and need.
Author: | Marcus Connor |
Created on: | NOVEMBER 8, 2018 |
Made with: | HTML, CSS |
Demo Link: | Source Code / Demo |
Tags: | Checkbox Rocker Toggle Switch |
If you liked this article Awesome Html Toggle Switch Examples, you should check out this one 25+ Input Radio Button Examples.