Hello guys, today I am going to show you How to build a JavaScript Random Quote Generator using HTML CSS & JavaScript, in this article, I will create a simple quote generator.
A Random Quote Generator is capable of pulling quotes randomly from an API or simply from an array. We will be designing a Random Quote Generator from scratch using HTML, CSS, JavaScript, in this article, we will generate quotes from the array using javascript.
JavaScript Random Quote Generator step by step
Step 1 — Creating a New Project
The first thing we’ll do is create a folder that will contain all of the files that make up the project. Create an empty folder on your devices and name it “Random Quote Generator”.
Open up Visual Studio Code or any Text editor, and create files(index.html, style.css, quotes.js) inside the folder. for creating a Random Quote Generator. In the next step, you will start creating the structure of the webpage.
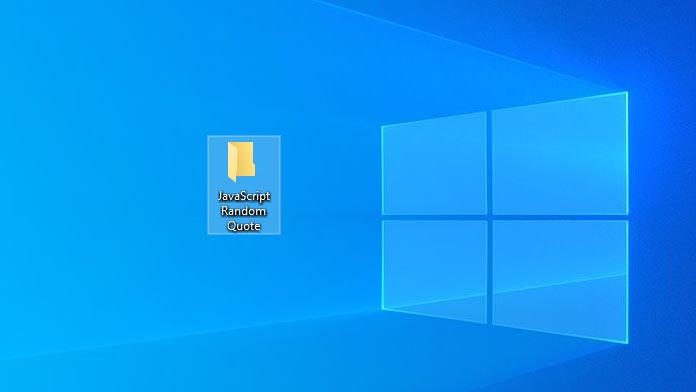
You may like these also:
Step 2 — Setting Up the basic structure
In this step, we will add the HTML code to create the basic structure of the project.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>How to build a JavaScript Random Quote Generator</title> <link rel="stylesheet" href="style.css"> <script src="quotes.js"></script> </head> <body> </body> </html>
This is the base structure of most web pages that use HTML.
Add the following code inside the <body>
tag:
<section class="randomQuote"> <header> <h2>Quote of the Day</h2> <p>Press the below button to receive a random quote!</p> </header> <div class="quotesOutput"> <p id="generatedQuote">"The greatest glory in living lies not in never falling, but in rising every time we fall."</p> <span id="AuthorName">--Nelson Mandela</span> </div> <button onclick="generateQoute()">New Quote</button> </section>
Step 3 — Adding Styles for the Classes
In this step, we will add styles to the section class Inside style.css file
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@300&display=swap'); * { padding: 0; margin: 0; font-family: 'Poppins', sans-serif; } header { text-align: center; background: #4b00ff; color: #fff; padding: 10px 0; margin-bottom: 10px; } .quotesOutput { text-align: center; background: #dde1ff; padding: 20px; min-height: 50px; margin-bottom: 20px; } button { display: block; width: 150px; height: 40px; font-size: 16px; font-weight: 600; background: #4b00ff; color: #fff; border: transparent; margin: auto; cursor: pointer; } p#generatedQuote { font-size: 16px; font-weight: 800; }
Step 4 — Adding some lines of JavaScript code
In this step, we will add some JavaScript code to build quote generator.
const arrayOfQuotes = [ {'author': 'Nelson Mandela', 'quote': 'The greatest glory in living lies not in never falling, but in rising every time we fall.' }, {'author': 'Walt Disney', 'quote': 'The way to get started is to quit talking and begin doing.' }, {'author': 'Eleanor Roosevelt', 'quote': 'If life were predictable it would cease to be life, and be without flavor.' }, {'author': 'Oprah Winfrey', 'quote': 'If you look at what you have in life, you`ll always have more. If you look at what you don`t have in life, you`ll never have enough' }, {'author': 'James Cameron', 'quote': 'If you set your goals ridiculously high and it`s a failure, you will fail above everyone else`s success' }, {'author': 'Elbert Hubbard', 'quote': 'Do not take life too seriously. You will not get out alive.' }, {'author': 'John Lennon', 'quote': 'Life is what happens when you`re busy making other plans.' } ]; // Create a function to generate quote from array function generateQoute(){ const random = Number.parseInt(Math.random()*arrayOfQuotes.length + 1); document.querySelector("#generatedQuote") .textContent = `\"${arrayOfQuotes[random].quote}\"`; document.querySelector("#AuthorName") .textContent = `--${arrayOfQuotes[random].author}`; }
Random Quote Generator Final Result
If you want source code you can download it from the below button
Random quote generator javascript codepen
#1 Random Quote Generator (HTML, CSS, JS)
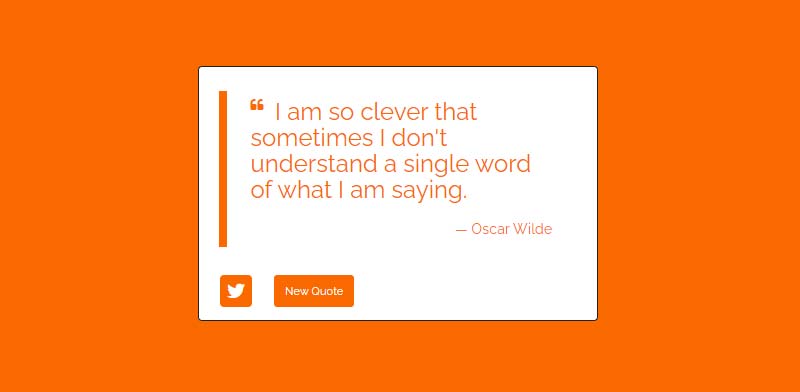
Simple quote generator using HTML CSS and JavaScript, which was developed by Alexander Lamm. Moreover, you can customize it according to your wish and need.
Author: | Alexander Lamm |
Created on: | February 4, 2016 |
Made with: | HTML CSS and JavaScript |
Demo Link: | Source Code / Demo |
Tags: | Simple quote generator |
#2 Ryan’s Random Quote Generator
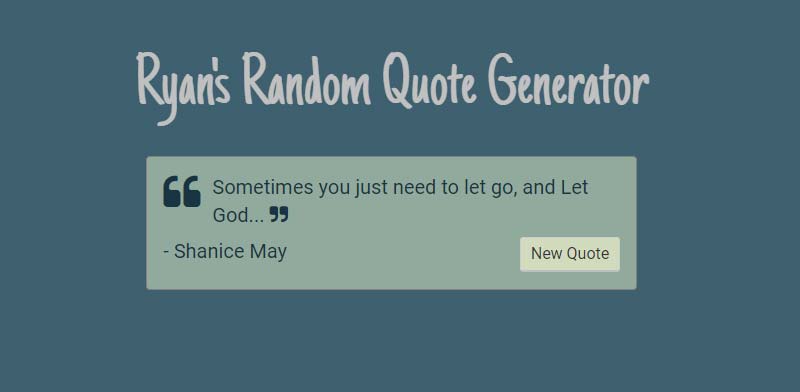
Simple random quote generator using HTML CSS and JavaScript, which was developed by Ryan. Moreover, you can customize it according to your wish and need.
Author: | Ryan |
Created on: | August 14, 2017 |
Made with: | HTML CSS and JavaScript |
Demo Link: | Source Code / Demo |
Tags: | basic quote generator |
#3 Simple Random Quote Generator
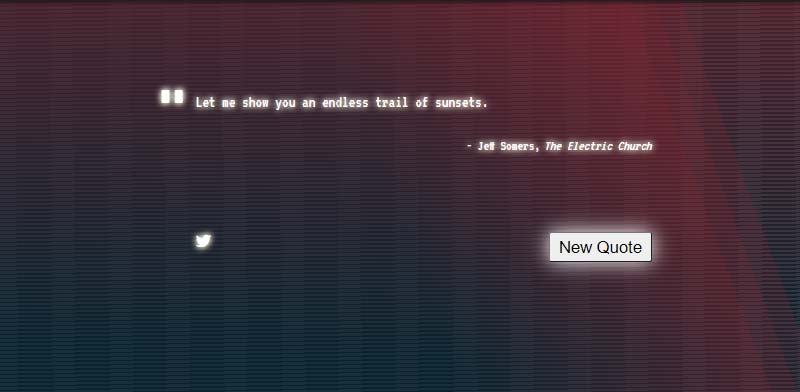
Awesome random quote generator with gradient background using HTML CSS and JavaScript, which was developed by Kenny Jeurissen. Moreover, you can customize it according to your wish and need.
Author: | Kenny Jeurissen |
Created on: | July 23, 2016 |
Made with: | HTML CSS and JavaScript |
Demo Link: | Source Code / Demo |
Tags: | unique quote generator |
We hope you liked this article. You should definitely keep your thoughts about it in the comment below and share this article with your friends.